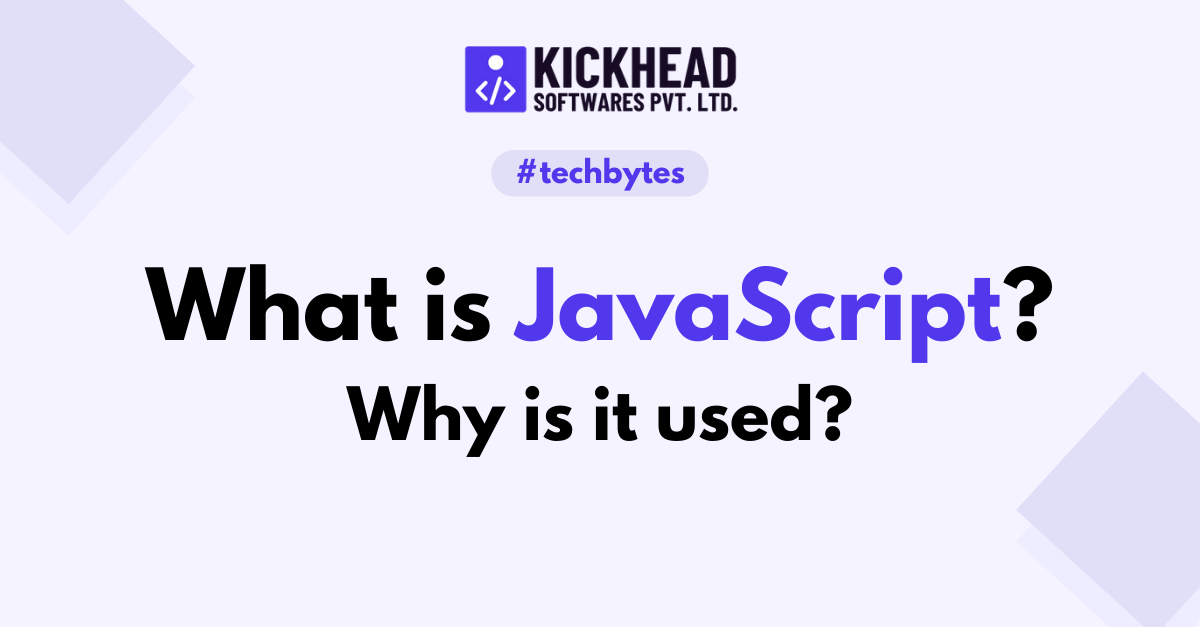
JavaScript is a programming language that is widely used in web development to create interactive and dynamic websites.
JavaScript is a programming language that is widely used in web development to create interactive and dynamic websites. It is a client-side scripting language, which means that the code is executed by the user's web browser, rather than on a server. This allows for faster and more responsive web pages, as the code is executed on the client-side rather than having to wait for a server response.
JavaScript is used to create a wide range of interactive and dynamic features on web pages, such as:
-
Form validation: JavaScript can be used to check that form fields have been filled out correctly before the form is submitted. For example, a script can check that an email address is in the correct format and that a password meets certain security requirements.
<form> <label for="email">Email:</label> <input type="email" id="email" name="email"/> <button type="submit" onclick="validateForm()">Submit</button> </form> <script> function validateForm() { var email = document.getElementById("email").value; if (!email.includes("@")) { alert("Please enter a valid email address"); } else { alert("Thanks for submitting!"); } } </script>
-
Image sliders: JavaScript can be used to create image sliders that automatically display a series of images, with the ability to navigate through them using next and previous buttons.
<div id="slider"> <img src="image1.jpg" id="current-img"> <button onclick="prevImage()">Prev</button> <button onclick="nextImage()">Next</button> </div> <script> var images = ["image1.jpg", "image2.jpg", "image3.jpg"]; var currentImageIndex = 0; function prevImage() { currentImageIndex = currentImageIndex > 0 ? currentImageIndex - 1 : images.length - 1; document.getElementById("current-img").src = images[currentImageIndex]; } function nextImage() { currentImageIndex = currentImageIndex < images.length - 1 ? currentImageIndex + 1 : 0; document.getElementById("current-img").src = images[currentImageIndex]; } </script>
-
Interactive maps: JavaScript can be used to create interactive maps that allow users to zoom in and out, pan around, and interact with map markers and other features.
-
Single-page applications: JavaScript is often used to create single-page applications (SPAs) that can run entirely in the browser, providing a more seamless user experience by dynamically updating the content on the page without requiring a full page reload.
-
Web-based games: JavaScript can be used to create web-based games, from simple arcade-style games to more complex multiplayer games.
JavaScript also allows communication with other technologies such as HTML, CSS, and web server technologies like Node.js, allowing for the creation of dynamic and interactive web pages.
In summary, JavaScript is a powerful and versatile programming language that is essential for creating interactive and dynamic web pages. It is widely supported by web browsers and is used by a large community of developers worldwide
Related Blogs
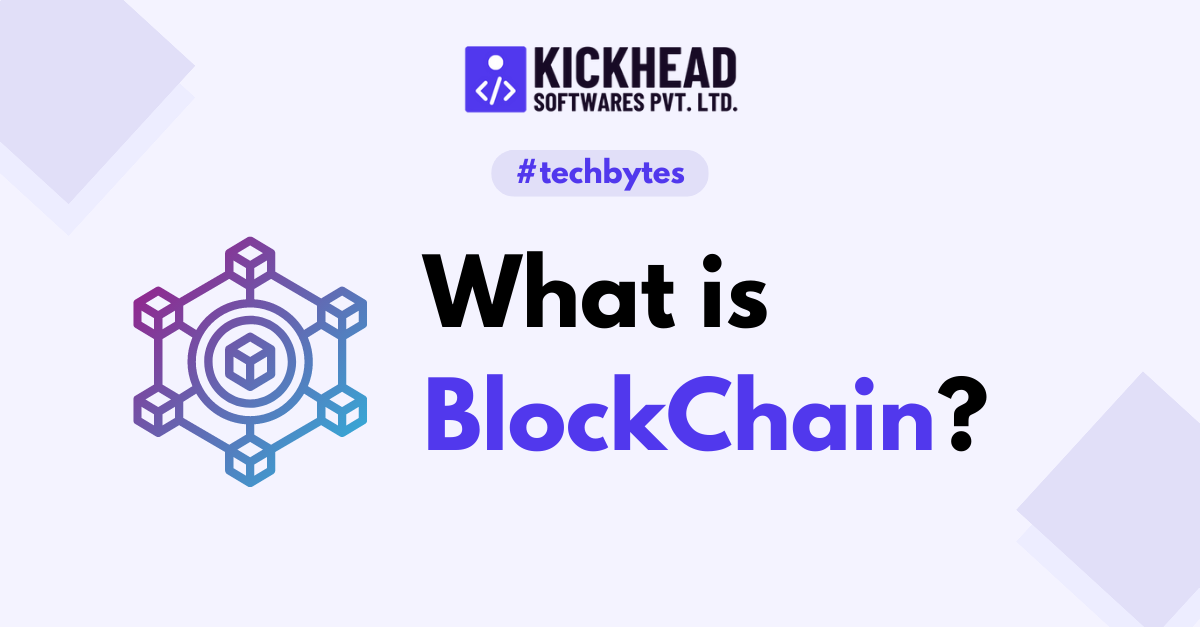